Check if key exists in hash
In Ruby on Rails Programming you might have to check if key exists in hash and based on that you might have to perform other operation. There is a simple way to detect if key exists in particular hash.
Ruby Hashes
Ruby hash contains Key-Value pairs. In Ruby, you can create a simple hash as follows,
my_hash = {}
Above code will initialize empty hash. Let’s add some key value pair in the my_hash
my_hash['name'] = 'Ruby in Rails'
The above code will add ‘name’ key and ‘Ruby in Rails’ as it’s value.
Let’s add one more key-value pair in the my_hash
my_hash['type'] = 'Ruby Programming Tutorials'
Now,
How to check if key exists in hash
You can check if particular key exists in a hash or not by using has_key? method available for the hash object in ruby.
Syntax | Description |
hash#has_key? sample |
This will return true/false by checking if key sample exists in hash object for which the method is invoked |
my_hash.has_key? 'name'
=> true
This will return true as name
key exists in the my_hash hash
my_hash.has_key? 'abc'
=> false
Above code returns false as my_hash doesn’t contain any key with named abc
Performance of Hash#has_key? versun Array#include?
Parameter | Hash#has_key? | Array#include |
---|---|---|
Time Complexity | o(1) operation | o(n) operation |
Access Type | Accesses Hash[key] if it returns any value then true is returned to the Hash#has_key? call |
Iterates through each element of the array till it finds the value in Array |
Conclusion
You can find more about ruby hashes and various methods that can be used with hashes on apidock. We learned how to check if key exists in a hash for Ruby language and compared it with include operation on arrays.
The has_key
is faster as it is o(1) lookup as explained above.
Subscribe to Ruby in Rails
Get the latest posts delivered right to your inbox
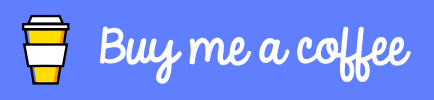