Rails ActiveSupport adds Enumerable#index_with method
Rails ActiveSupport Enumerable adds index_with method. This method allows creating hash with a value from block passed or default value. This is useful when we want to create a hash and value needs to be generated with some custom logic.
Background
- Rails Enumerable already has index_by which can be used to index elements of enumerable with a particular key.
- Enumerable#index_with comes in handy when we want a hash with customizable value.
Sample data
Let’s say we have a Post model as given below.
class Post < ApplicationRecord {
:id => :integer,
:title => :string,
:body => :integer
}
We have a post object as given below.
post = Post.first
# <Post:0x007fb9b024a218> {
# :id => 162973,
# :title => 'Enumerable index_with',
# :body => 'Use Enumerable#index_with to create hash with customizable value'
# }
Enumerable#index_with with a block
Now, we can use Enumerable#index_with
as given below.
%i(title body).index_with { |attribute| post.public_send(attribute) }
Output:
{
title: 'Enumerable index_with',
body: 'Use Enumerable#index_with to create hash with customizable value'
}
Enumerable#index_with with a deafult argument
index_with
used whatever passed to it as an argument as a value
for each key if block is not used.
Example 1
%i(title body).index_with(nil)
Output:
{
title: nil,
body: nil
}
Example 2
%i(title body).index_with([])
Output:
{
title: [],
body: []
}
Check out all the available methods on Enumerable to know more about handling Enumerable objects. If you want to try this feature out, compile with Rails master from Github.
Subscribe to Ruby in Rails
Get the latest posts delivered right to your inbox
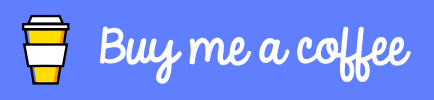